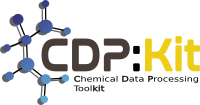 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BASE_CONTROLPARAMETERCONTAINER_HPP
30 #define CDPL_BASE_CONTROLPARAMETERCONTAINER_HPP
35 #include <unordered_map>
95 typedef std::unordered_map<LookupKey, Any, LookupKey::HashFunc> ParameterMap;
157 template <
typename T>
158 void setParameter(
const LookupKey& key,
T&& val);
198 template <
typename T>
199 const T& getParameter(
const LookupKey& key,
bool local =
false)
const;
218 template <
typename T>
219 const T& getParameterOrDefault(
const LookupKey& key,
const T& def_val,
bool local =
false)
const;
398 parameters(cntnr.parameters), parent(0) {}
419 void parameterRemoved(
const LookupKey&)
const;
420 void parameterChanged(
const LookupKey&,
const Any&)
const;
422 void parentParameterRemoved(
const LookupKey&)
const;
423 void parentParameterChanged(
const LookupKey&,
const Any&)
const;
425 void parentChanged()
const;
427 inline bool isEmptyAny(
const Any& val)
const;
429 template <
typename T>
430 bool isEmptyAny(
const T& val)
const;
432 typedef std::vector<ControlParameterContainer*> ChildContainer;
433 typedef std::pair<std::size_t, ParameterChangedCallbackFunction> ParamChangedCallbackContainerEntry;
434 typedef std::vector<ParamChangedCallbackContainerEntry> ParamChangedCallbackContainer;
435 typedef std::pair<std::size_t, ParameterRemovedCallbackFunction> ParamRemovedCallbackContainerEntry;
436 typedef std::vector<ParamRemovedCallbackContainerEntry> ParamRemovedCallbackContainer;
437 typedef std::pair<std::size_t, ParentChangedCallbackFunction> ParentChangedCallbackContainerEntry;
438 typedef std::vector<ParentChangedCallbackContainerEntry> ParentChangedCallbackContainer;
440 ParameterMap parameters;
442 mutable ChildContainer children;
443 ParamChangedCallbackContainer paramChangedCallbacks;
444 ParamRemovedCallbackContainer paramRemovedCallbacks;
445 ParentChangedCallbackContainer parentChangedCallbacks;
453 template <
typename T>
456 return getParameter(key,
true, local).template getData<T>();
459 template <
typename T>
462 const Any& val = getParameter(key,
false, local);
464 return (val.
isEmpty() ? def_val : val.template getData<T>());
467 template <
typename T>
470 if (isEmptyAny(val)) {
471 removeParameter(key);
475 const Any& any_val = (parameters[key] = std::forward<T>(val));
477 parameterChanged(key, any_val);
480 bool CDPL::Base::ControlParameterContainer::isEmptyAny(
const Any& val)
const
485 template <
typename T>
486 bool CDPL::Base::ControlParameterContainer::isEmptyAny(
const T&)
const
496 #endif // CDPL_BASE_CONTROLPARAMETERCONTAINER_HPP
ControlParameterContainer(const ControlParameterContainer &cntnr)
Constructs a copy of the ControlParameterContainer instance cntnr.
Definition: ControlParameterContainer.hpp:397
A class providing methods for the storage and lookup of control-parameter values.
Definition: ControlParameterContainer.hpp:93
bool isEmpty() const noexcept
Tells whether the Any instance stores any data.
Definition: Any.hpp:182
ConstParameterIterator getParametersEnd() const
Returns a constant iterator pointing to the end of the entries.
std::function< void()> ParentChangedCallbackFunction
A functor class that wraps callback target functions which get invoked when the parent container has ...
Definition: ControlParameterContainer.hpp:136
ConstParameterIterator getParametersBegin() const
Returns a constant iterator pointing to the beginning of the entries.
ParameterMap::const_iterator ConstParameterIterator
A constant iterator used to iterate over the control-parameter entries.
Definition: ControlParameterContainer.hpp:107
#define CDPL_BASE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setParameter(const LookupKey &key, T &&val)
Sets the value of the control-parameter specified by key to val.
Definition: ControlParameterContainer.hpp:468
An unique lookup key for control-parameter and property values.
Definition: LookupKey.hpp:54
Definition of the preprocessor macro CDPL_BASE_API.
ControlParameterContainer()
Constructs an empty ControlParameterContainer instance.
Definition: ControlParameterContainer.hpp:388
void unregisterParentChangedCallback(std::size_t id)
Unregisters the callback specified by id.
Definition of the class CDPL::Base::LookupKey.
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
bool removeParameter(const LookupKey &key)
Removes the entry for the control-parameter specified by key.
const T & getParameterOrDefault(const LookupKey &key, const T &def_val, bool local=false) const
Returns the value of the control-parameter specified by key as a const reference to an object of type...
Definition: ControlParameterContainer.hpp:460
virtual ~ControlParameterContainer()
Destructor.
ConstParameterIterator end() const
Returns a constant iterator pointing to the end of the entries.
ConstParameterIterator begin() const
Returns a constant iterator pointing to the beginning of the entries.
std::size_t registerParentChangedCallback(const ParentChangedCallbackFunction &func)
Registers a callback target function that gets invoked when the parent container has been changed or ...
std::size_t registerParameterChangedCallback(const ParameterChangedCallbackFunction &func)
Registers a callback target function that gets invoked when the value of a control-parameter has chan...
bool isParameterSet(const LookupKey &key, bool local=false) const
Tells whether or not a value has been assigned to the control-parameter specified by key.
std::function< void(const LookupKey &)> ParameterRemovedCallbackFunction
A functor class that wraps callback target functions which get invoked when a control-parameter entry...
Definition: ControlParameterContainer.hpp:125
std::size_t registerParameterRemovedCallback(const ParameterRemovedCallbackFunction &func)
Registers a callback target function that gets invoked when a control-parameter entry has been remove...
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
void unregisterParameterChangedCallback(std::size_t id)
Unregisters the callback specified by id.
void setParent(const ControlParameterContainer *cntnr)
Sets or removes the parent control-parameter container used to resolve requests for missing entries.
std::size_t getNumParameters() const
Returns the number of container entries.
The namespace of the Chemical Data Processing Library.
const ControlParameterContainer * getParent() const
Returns a pointer to the parent control-parameter container.
void unregisterParameterRemovedCallback(std::size_t id)
Unregisters the callback specified by id.
void copyParameters(const ControlParameterContainer &cntnr)
Replaces the current set of properties by a copy of the entries in cntnr.
const Any & getParameter(const LookupKey &key, bool throw_=false, bool local=false) const
Returns the value of the control-parameter specified by key.
ParameterMap::value_type ParameterEntry
A Base::LookupKey / Base::Any pair used to store the control-parameter values and associated keys.
Definition: ControlParameterContainer.hpp:102
ControlParameterContainer & operator=(const ControlParameterContainer &cntnr)
Assignment operator.
void clearParameters()
Erases all container entries.
const ControlParameterContainer & getParameters() const
Returns a const reference to itself.
Definition: ControlParameterContainer.hpp:491
Definition of the class CDPL::Base::Any.
std::function< void(const LookupKey &, const Any &)> ParameterChangedCallbackFunction
A functor class that wraps callback target functions which get invoked when the value of a control-pa...
Definition: ControlParameterContainer.hpp:116
void addParameters(const ControlParameterContainer &cntnr)
Adds the control-parameter value entries in the ControlParameterContainer instance cntnr.