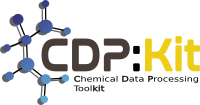 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_COMMONCONNECTEDSUBSTRUCTURESEARCH_HPP
30 #define CDPL_CHEM_COMMONCONNECTEDSUBSTRUCTURESEARCH_HPP
38 #include <boost/iterator/indirect_iterator.hpp>
64 typedef std::vector<AtomBondMapping*> ABMappingList;
67 typedef std::shared_ptr<CommonConnectedSubstructureSearch>
SharedPointer;
72 typedef boost::indirect_iterator<ABMappingList::iterator, AtomBondMapping>
MappingIterator;
77 typedef boost::indirect_iterator<ABMappingList::const_iterator, const AtomBondMapping>
ConstMappingIterator;
285 void initMatchExpressions();
287 bool findEquivAtoms();
288 bool findEquivBonds();
291 bool mapAtoms(std::size_t);
292 bool mapAtoms(std::size_t, std::size_t);
294 bool nextTargetAtom(std::size_t, std::size_t&, std::size_t&)
const;
298 bool hasPostMappingMatchExprs()
const;
301 bool foundMappingUnique();
304 void clearMappings();
306 void freeAtomBondMappings();
307 void freeAtomBondMapping();
315 void initQueryAtomMask(std::size_t);
316 void initTargetAtomMask(std::size_t);
318 void initQueryBondMask(std::size_t);
319 void initTargetBondMask(std::size_t);
321 void setQueryAtomBit(std::size_t);
322 void setTargetAtomBit(std::size_t);
324 void resetQueryAtomBit(std::size_t);
325 void resetTargetAtomBit(std::size_t);
327 bool testTargetAtomBit(std::size_t)
const;
329 void setQueryBondBit(std::size_t);
330 void setTargetBondBit(std::size_t);
332 void resetBondMasks();
334 bool operator<(
const ABMappingMask&)
const;
335 bool operator>(
const ABMappingMask&)
const;
346 typedef std::vector<Util::BitSet> BitMatrix;
347 typedef std::vector<const Atom*> AtomMappingTable;
348 typedef std::vector<std::size_t> AtomIndexList;
349 typedef std::vector<std::size_t> BondMappingStack;
350 typedef std::deque<std::size_t> AtomQueue;
351 typedef std::set<ABMappingMask> UniqueMappingList;
352 typedef std::vector<const Atom*> AtomList;
353 typedef std::vector<const Bond*> BondList;
354 typedef std::vector<MatchExpression<Atom, MolecularGraph>::SharedPointer> AtomMatchExprTable;
355 typedef std::vector<MatchExpression<Bond, MolecularGraph>::SharedPointer> BondMatchExprTable;
360 BitMatrix atomEquivMatrix;
361 BitMatrix bondEquivMatrix;
362 AtomQueue termQueryAtoms;
363 AtomIndexList termTargetAtoms;
364 BondMappingStack bondMappingStack;
365 AtomMappingTable queryAtomMapping;
366 ABMappingMask mappingMask;
370 ABMappingList foundMappings;
371 UniqueMappingList uniqueMappings;
372 AtomMatchExprTable atomMatchExprTable;
373 BondMatchExprTable bondMatchExprTable;
374 MolGraphMatchExprPtr molGraphMatchExpr;
375 AtomList postMappingMatchAtoms;
376 BondList postMappingMatchBonds;
377 MappingCache mappingCache;
382 bool maxMappingsOnly;
383 std::size_t numQueryAtoms;
384 std::size_t numQueryBonds;
385 std::size_t numTargetAtoms;
386 std::size_t numTargetBonds;
387 std::size_t numMappedAtoms;
388 std::size_t currMaxSubstructureSize;
389 std::size_t maxBondStackSize;
390 std::size_t maxNumMappings;
391 std::size_t minSubstructureSize;
396 #endif // CDPL_CHEM_COMMONCONNECTEDSUBSTRUCTURESEARCH_HPP
const AtomBondMapping & getMapping(std::size_t idx) const
Returns a const reference to the stored atom/bond mapping object at index idx.
std::size_t getNumMappings() const
Returns the number of atom/bond mappings that were recorded in the last search for common substructur...
MappingIterator begin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
Definition of the class CDPL::Util::ObjectStack.
void setMaxNumMappings(std::size_t max_num_mappings)
Allows to specify a limit on the number of stored atom/bond mappings.
bool uniqueMappingsOnly() const
Tells whether duplicate atom/bond mappings are discarded.
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
ConstMappingIterator end() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
MappingIterator getMappingsBegin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: CommonConnectedSubstructureSearch.hpp:77
bool findMaxMappings(const MolecularGraph &target)
Searches for all maximum-sized atom/bond mappings of connected query subgraphs to substructures of th...
AtomBondMapping & getMapping(std::size_t idx)
Returns a non-const reference to the stored atom/bond mapping object at index idx.
bool mappingExists(const MolecularGraph &target)
Searches for a common connected substructure between the query and the specified target molecular gra...
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
~CommonConnectedSubstructureSearch()
Destructor.
ConstMappingIterator getMappingsBegin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
std::size_t getMinSubstructureSize() const
Returns the minimum accepted common substructure size.
CommonConnectedSubstructureSearch.
Definition: CommonConnectedSubstructureSearch.hpp:62
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
void setQuery(const MolecularGraph &query)
Allows to specify a new query structure.
MappingIterator getMappingsEnd()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
Definition of the class CDPL::Chem::AtomBondMapping.
CommonConnectedSubstructureSearch()
Constructs and initializes a CommonConnectedSubstructureSearch instance.
MappingIterator end()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
ConstMappingIterator getMappingsEnd() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
void setMinSubstructureSize(std::size_t min_size)
Allows to specify the minimum accepted common substructure size.
std::size_t getMaxNumMappings() const
Returns the specified limit on the number of stored atom/bond mappings.
std::shared_ptr< CommonConnectedSubstructureSearch > SharedPointer
Definition: CommonConnectedSubstructureSearch.hpp:67
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
void uniqueMappingsOnly(bool unique)
Allows to specify whether or not to store only unique atom/bond mappings.
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: CommonConnectedSubstructureSearch.hpp:72
bool findAllMappings(const MolecularGraph &target)
Searches for all possible atom/bond mappings of connected query subgraphs to substructures of the spe...
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
CommonConnectedSubstructureSearch(const MolecularGraph &query)
Constructs and initializes a CommonConnectedSubstructureSearch instance for the specified query struc...
ConstMappingIterator begin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.