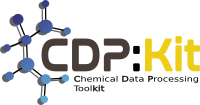 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_BONDFUNCTIONS_HPP
30 #define CDPL_CHEM_BONDFUNCTIONS_HPP
48 class StereoDescriptor;
129 bool check_term_n =
true,
bool check_order =
true, std::size_t min_ring_size = 8);
193 #endif // CDPL_CHEM_BONDFUNCTIONS_HPP
CDPL_CHEM_API const StereoDescriptor & getStereoDescriptor(const Atom &atom)
CDPL_CHEM_API bool getRingFlag(const Atom &atom)
CDPL_CHEM_API void clearStereoDescriptor(Atom &atom)
CDPL_CHEM_API bool isStereoCenter(const Atom &atom, const MolecularGraph &molgraph, bool check_asym=true, bool check_inv_n=true, bool check_quart_n=true, bool check_plan_n=true, bool check_amide_n=true, bool check_res_ctrs=true)
Definition of the preprocessor macro CDPL_CHEM_API.
Definition of the class CDPL::Math::VectorArray.
CDPL_CHEM_API void setReactionCenterStatus(Atom &atom, unsigned int status)
CDPL_CHEM_API void set2DStereoFlag(Bond &bond, unsigned int flag)
CDPL_CHEM_API void clearStereoCenterFlag(Atom &atom)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
CDPL_CHEM_API void setMatchExpression(Atom &atom, const MatchExpression< Atom, MolecularGraph >::SharedPointer &expr)
CDPL_CHEM_API bool has2DStereoFlag(const Bond &bond)
CDPL_CHEM_API unsigned int perceiveSybylType(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API void getContainingFragments(const Atom &atom, const FragmentList &frag_list, FragmentList &cont_frag_list, bool append=false)
std::shared_ptr< MatchConstraintList > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchConstraintList instances.
Definition: MatchConstraintList.hpp:197
CDPL_CHEM_API void clearMatchExpression(Atom &atom)
CDPL_CHEM_API bool hasStereoCenterFlag(const Atom &atom)
CDPL_CHEM_API bool hasMatchExpression(const Atom &atom)
CDPL_CHEM_API bool hasReactionCenterStatus(const Atom &atom)
CDPL_CHEM_API const std::string & getMatchExpressionString(const Atom &atom)
CDPL_CHEM_API bool hasDirection(const Bond &bond)
CDPL_CHEM_API MatchExpression< Atom, MolecularGraph >::SharedPointer generateMatchExpression(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API void setSybylType(Atom &atom, unsigned int type)
MolecularGraph.
Definition: MolecularGraph.hpp:52
CDPL_CHEM_API void clearSybylType(Atom &atom)
CDPL_CHEM_API void setRingFlag(Atom &atom, bool in_ring)
CDPL_CHEM_API std::size_t getOrder(const Bond &bond)
CDPL_CHEM_API unsigned int getSybylType(const Atom &atom)
CDPL_CHEM_API bool hasRingFlag(const Atom &atom)
CDPL_CHEM_API void clearMatchExpressionString(Atom &atom)
CDPL_CHEM_API unsigned int getCIPConfiguration(const Atom &atom)
CDPL_CHEM_API void clearDirection(Bond &bond)
CDPL_CHEM_API bool hasSybylType(const Atom &atom)
CDPL_CHEM_API bool hasMatchExpressionString(const Atom &atom)
CDPL_CHEM_API bool getStereoCenterFlag(const Atom &atom)
CDPL_CHEM_API void clearMatchConstraints(Atom &atom)
CDPL_CHEM_API unsigned int getDirection(const Bond &bond)
CDPL_CHEM_API void clearReactionCenterStatus(Atom &atom)
CDPL_CHEM_API void clearAromaticityFlag(Atom &atom)
CDPL_CHEM_API void setStereoCenterFlag(Atom &atom, bool is_center)
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
CDPL_CHEM_API std::size_t getNumContainingFragments(const Atom &atom, const FragmentList &frag_list)
CDPL_CHEM_API bool hasCIPConfiguration(const Atom &atom)
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
CDPL_CHEM_API void generateMatchExpressionString(const Atom &atom, const MolecularGraph &molgraph, std::string &expr_str)
CDPL_CHEM_API void setMatchExpressionString(Atom &atom, const std::string &expr_str)
CDPL_CHEM_API bool isInFragmentOfSize(const Atom &atom, const FragmentList &frag_list, std::size_t size)
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
CDPL_CHEM_API bool getAromaticityFlag(const Atom &atom)
CDPL_CHEM_API unsigned int get2DStereoFlag(const Bond &bond)
CDPL_CHEM_API void clear2DStereoFlag(Bond &bond)
CDPL_CHEM_API const MatchExpression< Atom, MolecularGraph >::SharedPointer & getMatchExpression(const Atom &atom)
CDPL_CHEM_API bool hasStereoDescriptor(const Atom &atom)
CDPL_CHEM_API bool hasOrder(const Bond &bond)
CDPL_CHEM_API void setAromaticityFlag(Atom &atom, bool aromatic)
CDPL_CHEM_API unsigned int calcConfiguration(const Atom &atom, const MolecularGraph &molgraph, const StereoDescriptor &descr, const Math::Vector3DArray &coords)
CDPL_CHEM_API unsigned int getReactionCenterStatus(const Atom &atom)
CDPL_CHEM_API bool hasAromaticityFlag(const Atom &atom)
A data structure for the storage and retrieval of stereochemical information about atoms and bonds.
Definition: StereoDescriptor.hpp:102
CDPL_CHEM_API void clearRingFlag(Atom &atom)
CDPL_CHEM_API void clearOrder(Bond &bond)
CDPL_CHEM_API void setMatchConstraints(Atom &atom, const MatchConstraintList::SharedPointer &constr)
CDPL_CHEM_API void setCIPConfiguration(Atom &atom, unsigned int config)
CDPL_CHEM_API void clearCIPConfiguration(Atom &atom)
CDPL_CHEM_API void setStereoDescriptor(Atom &atom, const StereoDescriptor &descr)
CDPL_CHEM_API bool hasMatchConstraints(const Atom &atom)
Definition of the class CDPL::Chem::MatchConstraintList.
Definition of the class CDPL::Chem::FragmentList.
CDPL_CHEM_API StereoDescriptor calcStereoDescriptor(const Atom &atom, const MolecularGraph &molgraph, std::size_t dim=1)
CDPL_CHEM_API void setDirection(Bond &bond, unsigned int dir)
CDPL_CHEM_API void setOrder(Bond &bond, std::size_t order)
CDPL_CHEM_API const MatchConstraintList::SharedPointer & getMatchConstraints(const Atom &atom)
CDPL_CHEM_API std::size_t getSizeOfLargestContainingFragment(const Atom &atom, const FragmentList &frag_list)
CDPL_CHEM_API std::size_t getSizeOfSmallestContainingFragment(const Atom &atom, const FragmentList &frag_list)