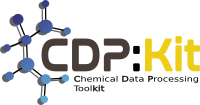 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ATOMFUNCTIONS_HPP
30 #define CDPL_CHEM_ATOMFUNCTIONS_HPP
34 #include <type_traits>
53 class StereoDescriptor;
269 bool check_inv_n =
true,
bool check_quart_n =
true,
bool check_plan_n =
true,
270 bool check_amide_n =
true,
bool check_res_ctrs =
true);
424 template <
typename AtomType,
typename OutputIterator>
427 typedef typename std::conditional<std::is_const<AtomType>::value,
typename AtomType::ConstAtomIterator,
typename AtomType::AtomIterator>::type AtomIterator;
429 AtomIterator atoms_end = atom.getAtomsEnd();
430 typename AtomType::ConstBondIterator b_it = atom.getBondsBegin();
431 std::size_t count = 0;
433 for (AtomIterator a_it = atom.getAtomsBegin(); a_it != atoms_end; ++a_it, ++b_it) {
434 if (&(*a_it) == excl_atom)
447 template <
typename AtomType,
typename OutputIterator>
450 typedef typename std::conditional<std::is_const<AtomType>::value,
typename AtomType::ConstBondIterator,
typename AtomType::BondIterator>::type BondIterator;
452 BondIterator bonds_end = atom.getBondsEnd();
453 typename AtomType::ConstAtomIterator a_it = atom.getAtomsBegin();
454 std::size_t count = 0;
456 for (BondIterator b_it = atom.getBondsBegin(); b_it != bonds_end; ++a_it, ++b_it) {
457 if (&(*a_it) == excl_atom)
470 template <
typename AtomType,
typename AtomOutputIterator,
typename BondOutputIterator>
473 typedef typename std::conditional<std::is_const<AtomType>::value,
typename AtomType::ConstAtomIterator,
typename AtomType::AtomIterator>::type AtomIterator;
474 typedef typename std::conditional<std::is_const<AtomType>::value,
typename AtomType::ConstBondIterator,
typename AtomType::BondIterator>::type BondIterator;
476 BondIterator bonds_end = atom.getBondsEnd();
477 AtomIterator a_it = atom.getAtomsBegin();
478 std::size_t count = 0;
480 for (BondIterator b_it = atom.getBondsBegin(); b_it != bonds_end; ++a_it, ++b_it) {
481 if (&(*a_it) == excl_atom)
498 #endif // CDPL_CHEM_ATOMFUNCTIONS_HPP
CDPL_CHEM_API const StereoDescriptor & getStereoDescriptor(const Atom &atom)
CDPL_CHEM_API bool getRingFlag(const Atom &atom)
CDPL_CHEM_API void clearHybridizationState(Atom &atom)
CDPL_CHEM_API unsigned int getTypeForSymbol(const Atom &atom)
CDPL_CHEM_API bool hasMOL2SubstructureChain(const Atom &atom)
CDPL_CHEM_API const std::string & getSymbol(const Atom &atom)
virtual bool containsBond(const Bond &bond) const =0
Tells whether the specified Chem::Bond instance is stored in this container.
CDPL_CHEM_API void clearStereoDescriptor(Atom &atom)
CDPL_CHEM_API void setFormalCharge(Atom &atom, long charge)
CDPL_CHEM_API bool isStereoCenter(const Atom &atom, const MolecularGraph &molgraph, bool check_asym=true, bool check_inv_n=true, bool check_quart_n=true, bool check_plan_n=true, bool check_amide_n=true, bool check_res_ctrs=true)
CDPL_CHEM_API void setCanonicalNumber(Atom &atom, std::size_t num)
CDPL_CHEM_API std::size_t getCanonicalNumber(const Atom &atom)
CDPL_CHEM_API void clearMOL2SubstructureID(Atom &atom)
std::size_t getConnectedAtomsAndBonds(AtomType &atom, const MolecularGraph &molgraph, AtomOutputIterator ao_it, BondOutputIterator bo_it, AtomType *excl_atom=0)
Definition: Chem/AtomFunctions.hpp:471
CDPL_CHEM_API bool has2DCoordinates(const Atom &atom)
Definition of the preprocessor macro CDPL_CHEM_API.
CDPL_CHEM_API std::size_t getUnpairedElectronCount(const Atom &atom)
CDPL_CHEM_API void clearIsotope(Atom &atom)
CDPL_CHEM_API unsigned int getGenericType(const Atom &atom)
Definition of the class CDPL::Math::VectorArray.
CDPL_CHEM_API void setReactionCenterStatus(Atom &atom, unsigned int status)
CDPL_CHEM_API const Math::Vector3DArray::SharedPointer & get3DCoordinatesArray(const Atom &atom)
CDPL_CHEM_API std::size_t getTopologicalDistance(const Atom &atom1, const Atom &atom2, const MolecularGraph &molgraph)
CDPL_CHEM_API void setHybridizationState(Atom &atom, unsigned int state)
CDPL_CHEM_API bool hasFormalCharge(const Atom &atom)
CDPL_CHEM_API void clearStereoCenterFlag(Atom &atom)
CDPL_CHEM_API const std::string & getMOL2Name(const Atom &atom)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
CDPL_CHEM_API std::size_t getIsotope(const Atom &atom)
CDPL_CHEM_API void setMDLStereoCareFlag(Atom &atom, bool flag)
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
CDPL_CHEM_API void setSymbol(Atom &atom, const std::string &symbol)
CDPL_CHEM_API void setRadicalType(Atom &atom, unsigned int type)
CDPL_CHEM_API void setMatchExpression(Atom &atom, const MatchExpression< Atom, MolecularGraph >::SharedPointer &expr)
CDPL_CHEM_API void get2DCoordinates(const AtomContainer &cntnr, Math::Vector2DArray &coords, bool append=false)
CDPL_CHEM_API void setMOL2SubstructureName(Atom &atom, const std::string &id)
CDPL_CHEM_API unsigned int perceiveSybylType(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API void getContainingFragments(const Atom &atom, const FragmentList &frag_list, FragmentList &cont_frag_list, bool append=false)
CDPL_CHEM_API unsigned int calcMDLParity(const Atom &atom, const MolecularGraph &molgraph)
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
std::shared_ptr< MatchConstraintList > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchConstraintList instances.
Definition: MatchConstraintList.hpp:197
CDPL_CHEM_API void clearMatchExpression(Atom &atom)
CDPL_CHEM_API void clearMOL2SubstructureSubtype(Atom &atom)
std::size_t getIncidentBonds(AtomType &atom, const MolecularGraph &molgraph, OutputIterator it, AtomType *excl_atom=0)
Definition: Chem/AtomFunctions.hpp:448
CDPL_CHEM_API bool hasStereoCenterFlag(const Atom &atom)
CDPL_CHEM_API bool hasMatchExpression(const Atom &atom)
CDPL_CHEM_API void clearMOL2SubstructureName(Atom &atom)
CDPL_CHEM_API std::size_t getAtomMappingID(const Atom &atom)
CDPL_CHEM_API bool hasReactionCenterStatus(const Atom &atom)
Atom.
Definition: Atom.hpp:52
Fragment.
Definition: Fragment.hpp:52
CDPL_CHEM_API void clearUnpairedElectronCount(Atom &atom)
CDPL_CHEM_API std::size_t getEnvironment(const Atom &atom, const MolecularGraph &molgraph, std::size_t max_dist, Fragment &env, bool append=false)
CDPL_CHEM_API const std::string & getMatchExpressionString(const Atom &atom)
CDPL_CHEM_API void setType(Atom &atom, unsigned int type)
CDPL_CHEM_API std::size_t getMOL2SubstructureID(const Atom &atom)
CDPL_CHEM_API void clearCanonicalNumber(Atom &atom)
CDPL_CHEM_API StereoDescriptor calcStereoDescriptorFromMDLParity(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API MatchExpression< Atom, MolecularGraph >::SharedPointer generateMatchExpression(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API void clearName(Atom &atom)
CDPL_CHEM_API void setSybylType(Atom &atom, unsigned int type)
CDPL_CHEM_API bool hasUnpairedElectronCount(const Atom &atom)
CDPL_CHEM_API void clear2DCoordinates(Atom &atom)
CDPL_CHEM_API bool hasRadicalType(const Atom &atom)
CDPL_CHEM_API bool hasMDLStereoCareFlag(const Atom &atom)
CDPL_CHEM_API void setImplicitHydrogenCount(Atom &atom, std::size_t count)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
CDPL_CHEM_API void clearSymbol(Atom &atom)
CDPL_CHEM_API std::size_t getComponentGroupID(const Atom &atom)
CDPL_CHEM_API void clearAtomMappingID(Atom &atom)
CDPL_CHEM_API void clearSybylType(Atom &atom)
CDPL_CHEM_API bool hasImplicitHydrogenCount(const Atom &atom)
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
CDPL_CHEM_API const std::string & getName(const Atom &atom)
CDPL_CHEM_API const std::string & getMOL2SubstructureChain(const Atom &atom)
CDPL_CHEM_API void setRingFlag(Atom &atom, bool in_ring)
CDPL_CHEM_API std::size_t getCIPPriority(const Atom &atom)
CDPL_CHEM_API unsigned int getHybridizationState(const Atom &atom)
CDPL_CHEM_API void clearFormalCharge(Atom &atom)
CDPL_CHEM_API bool hasAtomMappingID(const Atom &atom)
CDPL_CHEM_API void clear3DCoordinatesArray(Atom &atom)
CDPL_CHEM_API void setIsotope(Atom &atom, std::size_t isotope)
CDPL_CHEM_API unsigned int getSybylType(const Atom &atom)
CDPL_CHEM_API bool hasRingFlag(const Atom &atom)
CDPL_CHEM_API void clearMatchExpressionString(Atom &atom)
CDPL_CHEM_API void setMOL2Name(Atom &atom, const std::string &name)
CDPL_CHEM_API unsigned int getCIPConfiguration(const Atom &atom)
std::size_t getConnectedAtoms(AtomType &atom, const MolecularGraph &molgraph, OutputIterator it, AtomType *excl_atom=0)
Definition: Chem/AtomFunctions.hpp:425
CDPL_CHEM_API void clearMOL2Charge(Atom &atom)
CDPL_CHEM_API void clearMOL2Name(Atom &atom)
CDPL_CHEM_API bool hasSybylType(const Atom &atom)
CDPL_CHEM_API bool has3DCoordinatesArray(const Atom &atom)
CDPL_CHEM_API bool hasMatchExpressionString(const Atom &atom)
CDPL_CHEM_API unsigned int getMDLParity(const Atom &atom)
CDPL_CHEM_API void setMDLParity(Atom &atom, unsigned int parity)
CDPL_CHEM_API const Math::Vector3D & getConformer3DCoordinates(const Atom &atom, std::size_t conf_idx)
CDPL_CHEM_API void clearType(Atom &atom)
CDPL_CHEM_API const std::string & getMOL2SubstructureName(const Atom &atom)
CDPL_CHEM_API bool getStereoCenterFlag(const Atom &atom)
CDPL_CHEM_API void clearMatchConstraints(Atom &atom)
CDPL_CHEM_API void setUnpairedElectronCount(Atom &atom, std::size_t count)
CDPL_CHEM_API void clearSymmetryClass(Atom &atom)
CDPL_CHEM_API void setMOL2Charge(Atom &atom, double charge)
Type definition of a generic wrapper class for storing user-defined Chem::Atom 3D-coordinates functio...
CDPL_CHEM_API void clearMDLStereoCareFlag(Atom &atom)
CDPL_CHEM_API bool hasMDLParity(const Atom &atom)
CDPL_CHEM_API bool hasSymbol(const Atom &atom)
CDPL_CHEM_API void clearRadicalType(Atom &atom)
CDPL_CHEM_API void clearReactionCenterStatus(Atom &atom)
CDPL_CHEM_API void setMOL2SubstructureSubtype(Atom &atom, const std::string &subtype)
CDPL_CHEM_API void clearImplicitHydrogenCount(Atom &atom)
CDPL_CHEM_API void clearAromaticityFlag(Atom &atom)
CDPL_CHEM_API void setStereoCenterFlag(Atom &atom, bool is_center)
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
CDPL_CHEM_API std::size_t getNumContainingFragments(const Atom &atom, const FragmentList &frag_list)
CDPL_CHEM_API bool hasComponentGroupID(const Atom &atom)
CDPL_CHEM_API void clearCIPPriority(Atom &atom)
CDPL_CHEM_API unsigned int getType(const Atom &atom)
CDPL_CHEM_API bool hasCIPPriority(const Atom &atom)
CDPL_CHEM_API bool hasCIPConfiguration(const Atom &atom)
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
CDPL_CHEM_API void clearMOL2SubstructureChain(Atom &atom)
CDPL_CHEM_API void setCIPPriority(Atom &atom, std::size_t priority)
CDPL_CHEM_API bool hasMorganNumber(const Atom &atom)
CDPL_CHEM_API void generateMatchExpressionString(const Atom &atom, const MolecularGraph &molgraph, std::string &expr_str)
CDPL_CHEM_API void setMatchExpressionString(Atom &atom, const std::string &expr_str)
CDPL_CHEM_API bool hasMOL2SubstructureName(const Atom &atom)
CDPL_CHEM_API bool isInFragmentOfSize(const Atom &atom, const FragmentList &frag_list, std::size_t size)
CDPL_CHEM_API void setMOL2SubstructureChain(Atom &atom, const std::string &chain)
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
CDPL_CHEM_API bool getAromaticityFlag(const Atom &atom)
CDPL_CHEM_API bool hasMOL2Charge(const Atom &atom)
CDPL_CHEM_API long calcFormalCharge(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API long getFormalCharge(const Atom &atom)
CDPL_CHEM_API std::size_t getImplicitHydrogenCount(const Atom &atom)
CDPL_CHEM_API void setAtomMappingID(Atom &atom, std::size_t id)
CDPL_CHEM_API double getMOL2Charge(const Atom &atom)
CDPL_CHEM_API void clearMorganNumber(Atom &atom)
CDPL_CHEM_API bool hasName(const Atom &atom)
CDPL_CHEM_API std::size_t getMorganNumber(const Atom &atom)
CDPL_CHEM_API void setSymmetryClass(Atom &atom, std::size_t class_id)
CDPL_CHEM_API bool hasIsotope(const Atom &atom)
CDPL_CHEM_API const MatchExpression< Atom, MolecularGraph >::SharedPointer & getMatchExpression(const Atom &atom)
CDPL_CHEM_API bool hasStereoDescriptor(const Atom &atom)
CDPL_CHEM_API unsigned int perceiveHybridizationState(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API void setAromaticityFlag(Atom &atom, bool aromatic)
CDPL_CHEM_API unsigned int calcConfiguration(const Atom &atom, const MolecularGraph &molgraph, const StereoDescriptor &descr, const Math::Vector3DArray &coords)
CVector< double, 2 > Vector2D
A bounded 2 element vector holding floating point values of type double.
Definition: Vector.hpp:1632
CDPL_CHEM_API void clearComponentGroupID(Atom &atom)
CDPL_CHEM_API unsigned int getReactionCenterStatus(const Atom &atom)
CDPL_CHEM_API bool hasAromaticityFlag(const Atom &atom)
CDPL_CHEM_API void setName(Atom &atom, const std::string &name)
A data structure for the storage and retrieval of stereochemical information about atoms and bonds.
Definition: StereoDescriptor.hpp:102
virtual bool containsAtom(const Atom &atom) const =0
Tells whether the specified Chem::Atom instance is stored in this container.
CDPL_CHEM_API bool hasCanonicalNumber(const Atom &atom)
CDPL_CHEM_API void clearRingFlag(Atom &atom)
CDPL_CHEM_API bool hasMOL2SubstructureSubtype(const Atom &atom)
CDPL_CHEM_API void setMOL2SubstructureID(Atom &atom, std::size_t id)
CDPL_CHEM_API void setMatchConstraints(Atom &atom, const MatchConstraintList::SharedPointer &constr)
CDPL_CHEM_API std::size_t calcImplicitHydrogenCount(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API bool hasMOL2SubstructureID(const Atom &atom)
CDPL_CHEM_API std::size_t getSymmetryClass(const Atom &atom)
CDPL_CHEM_API void setCIPConfiguration(Atom &atom, unsigned int config)
CDPL_CHEM_API bool hasType(const Atom &atom)
CDPL_CHEM_API void clearCIPConfiguration(Atom &atom)
CDPL_CHEM_API void setStereoDescriptor(Atom &atom, const StereoDescriptor &descr)
CDPL_CHEM_API bool hasMatchConstraints(const Atom &atom)
Definition of the class CDPL::Chem::MatchConstraintList.
Definition of the class CDPL::Chem::FragmentList.
CDPL_CHEM_API StereoDescriptor calcStereoDescriptor(const Atom &atom, const MolecularGraph &molgraph, std::size_t dim=1)
CDPL_CHEM_API bool hasSymmetryClass(const Atom &atom)
CDPL_CHEM_API void markReachableAtoms(const Atom &atom, const MolecularGraph &molgraph, Util::BitSet &atom_mask, bool reset=true)
CDPL_CHEM_API void clearMDLParity(Atom &atom)
CDPL_CHEM_API void setMorganNumber(Atom &atom, std::size_t num)
CDPL_CHEM_API const std::string & getMOL2SubstructureSubtype(const Atom &atom)
CDPL_CHEM_API bool hasHybridizationState(const Atom &atom)
CDPL_CHEM_API void setComponentGroupID(Atom &atom, std::size_t id)
CDPL_CHEM_API const std::string & getSymbolForType(const Atom &atom)
CDPL_CHEM_API const MatchConstraintList::SharedPointer & getMatchConstraints(const Atom &atom)
CDPL_CHEM_API std::size_t getSizeOfLargestContainingFragment(const Atom &atom, const FragmentList &frag_list)
Definition of vector data types.
CDPL_CHEM_API bool hasMOL2Name(const Atom &atom)
CDPL_CHEM_API bool getMDLStereoCareFlag(const Atom &atom)
CDPL_CHEM_API void set2DCoordinates(AtomContainer &cntnr, const Math::Vector2DArray &coords)
CDPL_CHEM_API unsigned int getRadicalType(const Atom &atom)
CDPL_CHEM_API std::size_t getSizeOfSmallestContainingFragment(const Atom &atom, const FragmentList &frag_list)
CDPL_CHEM_API void set3DCoordinatesArray(Atom &atom, const Math::Vector3DArray::SharedPointer &coords_array)