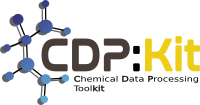 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
33 #ifndef CDPL_CHEM_CANONICALNUMBERINGCALCULATOR_HPP
34 #define CDPL_CHEM_CANONICALNUMBERINGCALCULATOR_HPP
74 static constexpr
unsigned int DEF_ATOM_PROPERTY_FLAGS =
82 static constexpr
unsigned int DEF_BOND_PROPERTY_FLAGS =
169 typedef std::vector<Edge*> EdgeList;
175 typedef std::vector<std::uint64_t> ConnectionTable;
183 void processNewSolution();
185 int testNewSolution();
187 void buildConnectionTable(ConnectionTable& ctab)
const;
188 void appendAtomConfigs(ConnectionTable& ctab);
189 void appendBondConfigs(ConnectionTable& ctab);
196 AtomNode* allocNode(
Calculator* calculator,
const Atom* atom, std::uint64_t label, std::size_t
id);
198 Edge* allocEdge(
const Calculator* calculator,
const Bond* bond, std::uint64_t label,
199 AtomNode* nbr_node, std::size_t
id);
205 typedef EdgeList::const_iterator EdgeIterator;
209 void init(
Calculator* calculator,
const Atom* atom, std::uint64_t label, std::size_t
id);
211 const Atom* getAtom()
const;
213 void addEdge(Edge* edge);
215 std::uint64_t getLabel()
const;
217 void setLabel(std::uint64_t label);
218 void setNewLabel(std::size_t label);
222 std::size_t getID()
const;
226 std::size_t getNumEdges()
const;
228 EdgeIterator getEdgesBegin()
const;
229 EdgeIterator getEdgesEnd()
const;
231 void appendConnectivityData(ConnectionTable& ctab)
const;
232 void appendBondConfigData(ConnectionTable& ctab)
const;
233 void appendAtomConfigData(ConnectionTable& ctab);
235 bool involvedInStereocenter();
237 bool isEquivalent(
const AtomNode* node)
const;
238 bool isNonEquivalent(
const AtomNode* node)
const;
240 void addToEquivalenceSet(
const AtomNode* node);
241 void addToNonEquivalenceSet(
const AtomNode* node);
243 static bool terminalAndOnCommonNonStereoNode(
const AtomNode* node1,
const AtomNode* node2);
252 bool initConfigurationData();
256 std::uint64_t initialLabel;
258 std::size_t newLabel;
263 bool hasConfiguration;
264 bool configDataValid;
265 bool partOfStereocenter;
266 bool partOfStereocenterValid;
275 void init(
const Calculator* calculator,
const Bond* bond, std::uint64_t label,
276 AtomNode* nbr_node, std::size_t
id);
278 void appendBondData(ConnectionTable&)
const;
279 void appendConfigurationData(
const AtomNode* node, ConnectionTable& ctab);
281 AtomNode* getNeighborNode()
const;
283 bool representsStereoBond(
const AtomNode* node);
285 std::size_t getID()
const;
294 bool initConfigurationData(
const AtomNode* node);
302 bool hasConfiguration;
303 bool configDataValid;
306 typedef std::pair<const Fragment*, const ConnectionTable*> CanonComponentInfo;
308 struct ComponentCmpFunc
311 bool operator()(
const CanonComponentInfo&,
const CanonComponentInfo&)
const;
314 typedef std::pair<AtomNode*, std::uint64_t> NodeLabelingState;
315 typedef std::vector<NodeLabelingState> NodeLabelingStack;
316 typedef std::vector<AtomNode*> NodeList;
317 typedef std::vector<ConnectionTable> ConnectionTableList;
318 typedef std::vector<CanonComponentInfo> CanonComponentList;
324 unsigned int atomPropertyFlags;
325 unsigned int bondPropertyFlags;
326 HydrogenCountFunction hCountFunc;
327 bool foundStereogenicAtoms;
328 bool foundStereogenicBonds;
333 NodeList equivNodeStack;
334 NodeLabelingStack nodeLabelingStack;
335 ConnectionTableList compConnectionTables;
336 ConnectionTableList levelConnectionTables;
337 ConnectionTable testConnectionTable;
338 NodeList minNodeList;
339 CanonComponentList canonComponentList;
345 #endif // CDPL_CHEM_CANONICALNUMBERINGCALCULATOR_HPP
Definition of the class CDPL::Util::ObjectStack.
const unsigned int FORMAL_CHARGE
Specifies the formal charge of an atom.
Definition: Chem/AtomPropertyFlag.hpp:73
const unsigned int AROMATICITY
Specifies the membership of a bond in aromatic rings.
Definition: BondPropertyFlag.hpp:73
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
const unsigned int CONFIGURATION
Specifies the steric configuration of a double bond.
Definition: BondPropertyFlag.hpp:78
const HydrogenCountFunction & getHydrogenCountFunction()
void calculate(const MolecularGraph &molgraph, Util::STArray &numbering)
Performs a canonical numbering of the atoms in the molecular graph molgraph.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
void setHydrogenCountFunction(const HydrogenCountFunction &func)
Definition: CanonicalNumberingCalculator.hpp:288
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Definition of the class CDPL::Util::Array.
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
const unsigned int CONFIGURATION
Specifies the configuration of a stereogenic atom.
Definition: Chem/AtomPropertyFlag.hpp:98
CanonicalNumberingCalculator(const MolecularGraph &molgraph, Util::STArray &numbering)
Constructs the CanonicalNumberingCalculator instance and performs a canonical numbering of the atoms ...
bool operator()(const Edge *, const Edge *) const
const unsigned int ORDER
Specifies the order of a bond.
Definition: BondPropertyFlag.hpp:63
void setBondPropertyFlags(unsigned int flags)
Allows to specify the set of bond properties that has to be considered by the canonical numering algo...
const unsigned int TYPE
Specifies the generic type or element of an atom.
Definition: Chem/AtomPropertyFlag.hpp:63
const unsigned int ISOTOPE
Specifies the isotopic mass of an atom.
Definition: Chem/AtomPropertyFlag.hpp:68
unsigned int getAtomPropertyFlags() const
Returns the set of atomic properties that gets considered by the canonical numbering algorithm.
CanonicalNumberingCalculator.
Definition: CanonicalNumberingCalculator.hpp:67
The namespace of the Chemical Data Processing Library.
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
void setAtomPropertyFlags(unsigned int flags)
Allows to specify the set of atomic properties that has to be considered by the canonical numering al...
A data structure for the storage and retrieval of stereochemical information about atoms and bonds.
Definition: StereoDescriptor.hpp:102
const unsigned int AROMATICITY
Specifies the membership of an atom in aromatic rings.
Definition: Chem/AtomPropertyFlag.hpp:93
Definition of the type CDPL::Chem::StereoDescriptor.
Definition: CanonicalNumberingCalculator.hpp:246
const unsigned int H_COUNT
Specifies the hydrogen count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:78
std::function< std::size_t(const Atom &, const MolecularGraph &)> HydrogenCountFunction
Definition: CanonicalNumberingCalculator.hpp:85
Definition of constants in namespace CDPL::Chem::BondPropertyFlag.
CanonicalNumberingCalculator()
Constructs the CanonicalNumberingCalculator instance.
bool operator()(const AtomNode *, const AtomNode *) const
unsigned int getBondPropertyFlags() const
Returns the set of bond properties that gets considered by the canonical numbering algorithm.
CDPL_CHEM_API void canonicalize(MolecularGraph &molgraph, const AtomCompareFunction &func, bool atoms=true, bool atom_nbrs=true, bool bonds=true, bool bond_atoms=false)