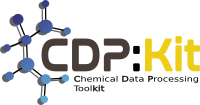 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_CAIRORENDERER2D_HPP
30 #define CDPL_VIS_CAIRORENDERER2D_HPP
84 void drawLine(
double x1,
double y1,
double x2,
double y2);
88 void drawText(
double x,
double y,
const std::string& txt);
89 void drawEllipse(
double x,
double y,
double width,
double height);
96 void fillPath()
const;
97 void strokePath()
const;
99 typedef std::vector<Pen> PenStack;
100 typedef std::vector<Brush> BrushStack;
101 typedef std::vector<Font> FontStack;
105 BrushStack brushStack;
111 #endif // CDPL_VIS_CAIRORENDERER2D_HPP
void setClipPath(const Path2D &path)
An interface that provides methods for low level 2D drawing operations.
Definition: Renderer2D.hpp:86
void drawText(double x, double y, const std::string &txt)
Draws the specified text at the position (x, y).
void drawRectangle(double x1, double y1, double x2, double y2)
Draws an axis aligned rectangle of the specified width and height whose upper-left corner is located ...
Definition of the class CDPL::Vis::Font.
Specifies how to draw lines and outlines of shapes.
Definition: Pen.hpp:53
void drawPoint(double x, double y)
Draws a point at the position (x, y).
void setBrush(const Brush &brush)
Sets the brush to be used in subsequent drawing operations.
void saveState()
Saves the current renderer state.
void setTransform(const Math::Matrix3D &xform)
Sets the applied affine transformation matrix to xform.
void drawPolyline(const Math::Vector2DArray &points)
Draws the polyline defined by points.
Implements the Renderer2D interface on top of the Cairo 2D Graphics Library.
Definition: CairoRenderer2D.hpp:57
struct _cairo cairo_t
Definition: CairoPointer.hpp:35
void setFont(const Font &font)
Sets the font to be used in subsequent text drawing operations.
Specifies a font for drawing text.
Definition: Font.hpp:54
Definition of the class CDPL::Vis::Pen.
Provides a container for painting operations, enabling arbitrary graphical shapes to be constructed a...
Definition: Path2D.hpp:78
void clearClipPath()
Disables clipping.
void drawLineSegments(const Math::Vector2DArray &points)
Draws the sequence of disjoint line segments defined by points.
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setPen(const Pen &pen)
Sets the pen to be used in subsequent drawing operations.
void drawEllipse(double x, double y, double width, double height)
Draws an ellipse with the given width and height around the center position (x, y).
CairoRenderer2D(const CairoPointer< cairo_t > &cairo_ctxt)
Constructs a renderer object that operates on the Cairo cdrawing ontext specified by cairo_ctxt.
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
Definition of the class CDPL::Vis::Renderer2D.
void drawLine(double x1, double y1, double x2, double y2)
Draws a line segment from (x1, y1) to (x2, y2).
void restoreState()
Restores the last renderer state saved by a call to saveState().
The namespace of the Chemical Data Processing Library.
void drawPath(const Path2D &path)
Draws the given path.
~CairoRenderer2D()
Destructor.
Specifies the fill pattern and fill color of shapes.
Definition: Brush.hpp:50
Definition of the class CDPL::Vis::Brush.
VectorArray< Vector2D > Vector2DArray
An array of Math::Vector2D objects.
Definition: VectorArray.hpp:79
void drawPolygon(const Math::Vector2DArray &points)
Draws the polygon defined by points.
Definition of the preprocessor macro CDPL_VIS_API.
Definition of the class CDPL::Vis::CairoPointer.
void transform(const Math::Matrix3D &xform)
Multiplies the current affine transformation matrix by xform.