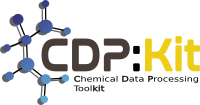 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ATOMDICTIONARY_HPP
30 #define CDPL_CHEM_ATOMDICTIONARY_HPP
35 #include <unordered_map>
39 #include <boost/iterator/transform_iterator.hpp>
40 #include <boost/functional/hash.hpp>
69 Entry(
unsigned int atom_type, std::size_t iso,
const std::string& sym,
70 const std::string& name, std::size_t most_abdt_iso,
double avg_weight,
71 std::size_t iupac_grp, std::size_t period,
bool metal,
bool non_metal,
const Util::STArray& val_states,
72 double vdw_rad,
const double cov_radii[3],
double ar_eneg,
const IsotopeMassMap& iso_masses);
105 unsigned int atomType;
109 std::size_t mostAbundantIso;
111 std::size_t iupacGroup;
117 double covalentRadii[3];
118 double allredRochowEneg;
123 typedef std::unordered_map<std::pair<unsigned int, std::size_t>,
Entry, boost::hash<std::pair<unsigned int, std::size_t> > > EntryLookupTable;
124 typedef std::unordered_map<std::string, unsigned int> SymbolToTypeLookupTable;
129 typedef boost::transform_iterator<std::function<
const Entry&(
const EntryLookupTable::value_type&)>,
130 EntryLookupTable::const_iterator>
170 static const std::string&
getSymbol(
unsigned int type, std::size_t isotope = 0);
184 static const std::string&
getName(
unsigned int type, std::size_t isotope = 0);
194 static unsigned int getType(
const std::string& symbol,
bool strict =
false);
336 EntryLookupTable entries;
337 SymbolToTypeLookupTable strictSymToTypeTable;
338 SymbolToTypeLookupTable nonstrictSymToTypeTable;
343 #endif // CDPL_CHEM_ATOMDICTIONARY_HPP
const std::string & getName() const
std::size_t getPeriod() const
static unsigned int getType(const std::string &symbol, bool strict=false)
Returns the numeric atom type that is associated with the specified atom type symbol.
Definition of the preprocessor macro CDPL_CHEM_API.
std::size_t getIUPACGroup() const
static std::size_t getIUPACGroup(unsigned int type)
Returns the IUPAC group of the chemical element specified by type.
bool containsEntry(unsigned int type, std::size_t isotope) const
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
static bool isNobleGas(unsigned int type)
Tells whether the chemical element specified by type is a noble gas.
static bool isHalogen(unsigned int type)
Tells whether the chemical element specified by type is a halogen.
const std::string & getSymbol() const
void removeEntry(unsigned int type, std::size_t isotope)
Definition of the class CDPL::Util::Map.
ConstEntryIterator begin() const
static bool isMetal(unsigned int type)
Tells whether the chemical element specified by type is a metal.
std::shared_ptr< AtomDictionary > SharedPointer
Definition: AtomDictionary.hpp:127
Definition of the class CDPL::Util::Array.
static double getAtomicWeight(unsigned int type, std::size_t isotope=0)
Returns the standard atomic weight or the relative isotopic mass of an isotope of the chemical elemen...
std::size_t getIsotope() const
static bool isNonMetal(unsigned int type)
Tells whether the chemical element specified by type is a non-metal.
static bool isTransitionMetal(unsigned int type)
Tells whether the chemical element specified by type is a transition metal.
double getAllredRochowElectronegativity() const
static const std::string & getName(unsigned int type, std::size_t isotope=0)
Returns the name of the chemical element that is associated with the specified atom type and isotope.
const Util::STArray & getValenceStates() const
double getAverageWeight() const
double getVdWRadius() const
static bool isChemicalElement(unsigned int type)
Tells whether the specified atom type represents a chemical element.
ConstEntryIterator getEntriesBegin() const
double getCovalentRadius(std::size_t order) const
static std::size_t getMostAbundantIsotope(unsigned int type)
Returns the mass number of the most abundant isotope of the chemical element specified by type.
static double getVdWRadius(unsigned int type)
Returns the van der Waals radius of the chemical element specified by type.
static bool isSemiMetal(unsigned int type)
Tells whether the chemical element specified by type is a semi-metal.
void addEntry(const Entry &entry)
ConstEntryIterator getEntriesEnd() const
unsigned int getType() const
Util::Map< std::size_t, double > IsotopeMassMap
Definition: AtomDictionary.hpp:65
The namespace of the Chemical Data Processing Library.
static const std::string & getSymbol(unsigned int type, std::size_t isotope=0)
Returns the symbol that is associated with the specified atom type and isotope.
boost::transform_iterator< std::function< const Entry &(const EntryLookupTable::value_type &)>, EntryLookupTable::const_iterator > ConstEntryIterator
Definition: AtomDictionary.hpp:131
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
std::size_t getNumEntries() const
static double getAllredRochowElectronegativity(unsigned int type)
Returns the Allred-Rochow electronegativity of the chemical element specified by type.
Definition: AtomDictionary.hpp:62
const Entry & getEntry(unsigned int type, std::size_t isotope) const
static void set(const SharedPointer &dict)
std::size_t getMostAbundantIsotope() const
A global dictionary for the lookup of data associated with the atom types defined in namespace Chem::...
Definition: AtomDictionary.hpp:58
static std::size_t getNumValenceElectrons(unsigned int type)
Returns the number of valence electrons of the chemical element specified by type.
static bool isMainGroupElement(unsigned int type)
Tells whether the chemical element specified by type is a main group element.
static const Util::STArray & getValenceStates(unsigned int type)
Returns an array with the valence states of the chemical element specified by type.
const IsotopeMassMap & getIsotopeMasses() const
static double getCovalentRadius(unsigned int type, std::size_t order=1)
Returns the covalent radius of the chemical element specified by type for the given bond order.
static std::size_t getPeriod(unsigned int type)
Returns the period number of the chemical element specified by type.
static const SharedPointer & get()
ConstEntryIterator end() const
Entry(unsigned int atom_type, std::size_t iso, const std::string &sym, const std::string &name, std::size_t most_abdt_iso, double avg_weight, std::size_t iupac_grp, std::size_t period, bool metal, bool non_metal, const Util::STArray &val_states, double vdw_rad, const double cov_radii[3], double ar_eneg, const IsotopeMassMap &iso_masses)